Mastering API Data Fetching with Combine in iOS: A Complete Guide
In this article, we will learn how to fetch posts from a remote API using SwiftUI and Combine. Before jumping into the code, let’s first take a moment to understand the key features of Combine and how it enhances our app.
What is Combine?
Combine is a framework in Swift that provides a declarative Swift API for processing values over time. It allows you to work with asynchronous events, such as network requests, and manage them with a functional programming style. Combine is used to work with streams of values, handle asynchronous operations, and manage state changes in a declarative way.
Some key features of Combine:
• Publishers: These represent a stream of values or events. A publisher emits values, which can be observed and acted upon.
• Subscribers: These listen for values emitted by a publisher. A subscriber can react to changes by executing specific actions.
• Operators: Combine provides a wide variety of operators (like map, filter, merge, decode, etc.) that allow you to transform, filter, or combine values as they are emitted.
• Cancellables: A cancellable represents a subscription to a publisher. It allows you to cancel the subscription to stop receiving values.
• Declarative and Reactive Programming: Combine enables a more declarative, functional approach to handling asynchronous data flows, making the code easier to read and maintain.
How Combine Works in Our App
In this example, we are going to fetch posts from a remote API and display them in a SwiftUI list. Combine will handle the network request, process the data asynchronously, and update the UI with the results. We will break this down step by step:
Step 1: Define the Model
First, we define a PostModel struct that will represent the data we will be fetching from the API. This model conforms to Identifiable and Codable so that it can be used in a SwiftUI List and be easily decoded from JSON.
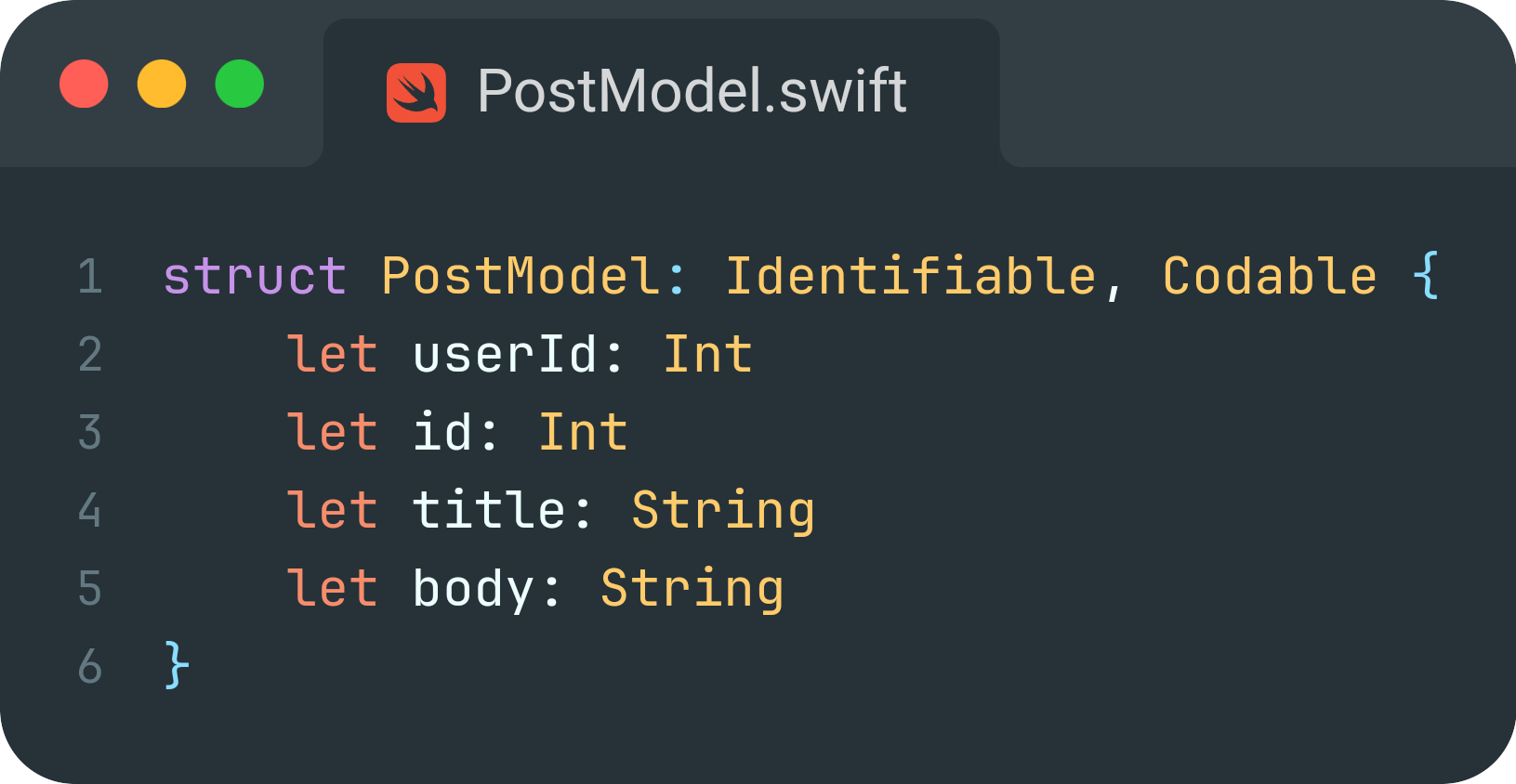
• Identifiable: Ensures each post has a unique identifier, useful for displaying in a list.
• Codable: Makes the model capable of being encoded and decoded, allowing us to convert between JSON and our PostModel easily.
Step 2: Create the ViewModel
The next step is creating a PostFetcherViewModel class, which will be responsible for making the API request using Combine. The view model will use Combine to fetch the posts asynchronously and update the UI.
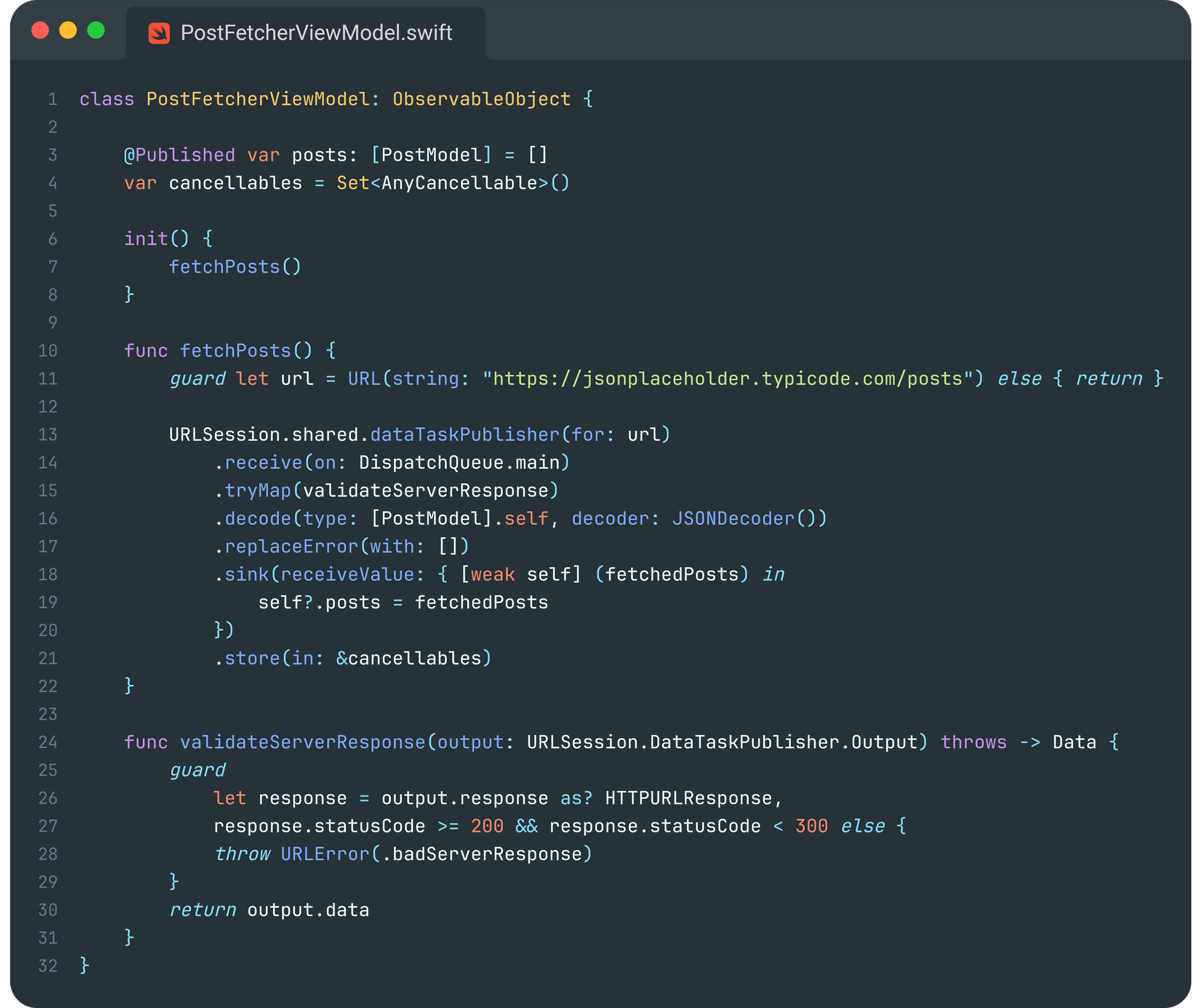
Key Concepts Explained:
• @Published: This property wrapper automatically notifies the view when the posts array is updated, triggering a UI update.
• fetchPosts(): This method performs the network request:
- dataTaskPublisher(for:): Creates a publisher that sends data from a URL.
- receive(on: DispatchQueue.main): Ensures that any updates to the UI happen on the main thread, which is essential in SwiftUI.
- tryMap(validateServerResponse): Verifies that the server response is valid. If not, it throws an error.
- decode(type: [PostModel].self, decoder: JSONDecoder()): Decodes the raw data into an array of PostModel instances.
- replaceError(with: []): If an error occurs, we replace the posts array with an empty array.
- sink(receiveValue:): This is the subscriber that handles the decoded posts and updates the posts array.
- store(in: &cancellables): Stores the subscription in a cancellables set, which is necessary for managing the lifecycle of the subscription.
Step 3: Create the SwiftUI View
Now we need a SwiftUI view to display the posts. We'll use a List to show each post’s title and body.
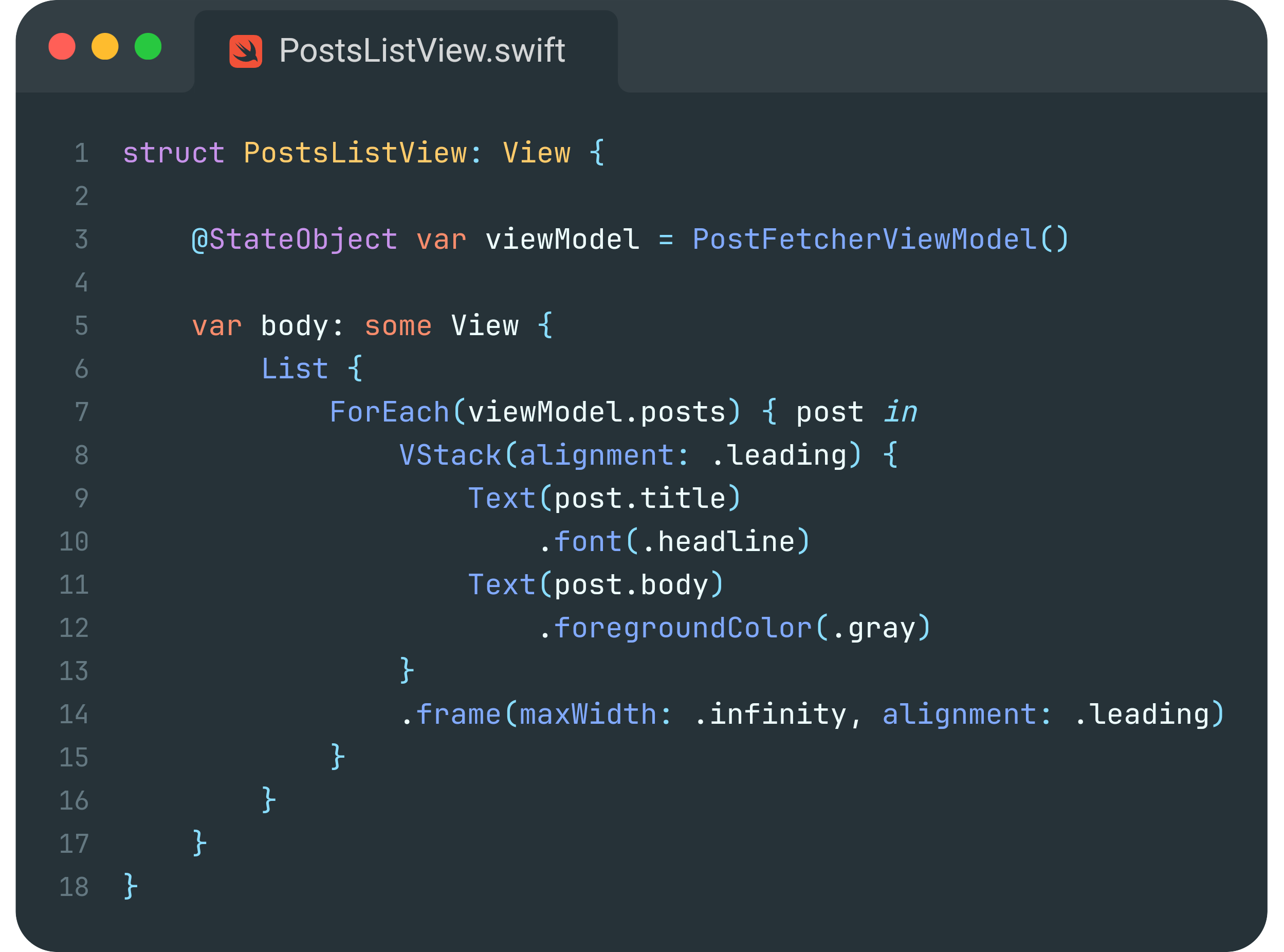
Explanation:
- @StateObject: This initializes the PostFetcherViewModel and ensures it persists during the view’s lifecycle. It is used to manage the view model's state in the view.
- List: The List component displays the PostModel data. We iterate over the viewModel.posts array using ForEach and display the post's title and body.
Conclusion
Now that you understand the key concepts of Combine, we’ve implemented a simple app that fetches data from an API and displays it using SwiftUI. The declarative nature of Combine makes handling asynchronous events smooth and clean, especially when paired with SwiftUI’s reactive framework. The combination of Publisher, Subscriber, and operators like decode, tryMap, and replaceError allows us to handle network requests, transform data, and manage errors efficiently.By mastering Combine, you can significantly improve your app development process, making your code more readable, maintainable, and scalable. I hope this guide helps you better understand how to integrate Combine with SwiftUI for handling asynchronous data fetching.If you found this guide helpful, please consider supporting my work by clapping, sharing it with your network, or leaving a comment below! Your feedback is incredibly valuable and motivates me to continue creating more content for the developer community.
Thank you for reading!❤️